A string is described as a collection of characters. String objects are immutable in Java, which means they can’t be modified once they’ve been created. A popular question asked in Java interviews is how to find the substring of a string. So, I’ll show you how Substring functions in Java.
We’ll be covering the following topics in this tutorial:
Substring in Java: What is a substring in Java?
Programmers often need to retrieve an individual character or a group of characters (substring) from a string. For example, in a word processing program, a part of the string is copied or deleted. While charAt()
method returns only a single character from a string, the substring()
method in the String class can be used to obtain a substring from a string.
Substring in Java: Different methods under substring
There are two separate methods under the substring() method. They are as follows:
• String substring(int begIndex)
• String substring(int beginIndex, int endIndex)
String substring(int beginIndex): This method returns a new string that is a substring of the invoking string. The substring returned contains a copy of characters beginning from the specified index beginIndex
and extends to the end of the string. For example,
Syntax:
public String substring(int begIndex)
Note: The index begins with ‘0,’ which corresponds to String’s first character.
Let’s take a look at an example.
s1.substring(3); // returns substring 'come'
String substring (int beginIndex, int endIndex): This method is another version of previous method. It also returns a substring that begins at the specified begin Index and extends to the character at index endIndex-1
.
Syntax:
public String substring(int begIndex, int endIndex)
Let’s take a look at an example.
s1.substring(3,6); // returns substring "come".
public class SubstringMethods { public static void main(String[] args) { String s1 = "Welcome" ; System.out.println("s1.substring(3) = " + s1.substring(3)); System.out.println("substring(3,6) = " + s1.substring(3)); } }
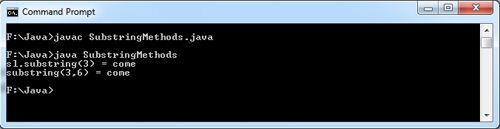
class StrSubString { public static void main(String args[]) { String k="Hello Dinesh"; String m=""; m = k.substring(6,12); System.out.println(m); } }