A pointer in C++ is a variable that contains the address of another variable in memory. Suppose i is an integer variable having value 10 stored at address 2202 and the address of i is assigned to variable j, then j is a pointer variable pointing to the memory address stored in it. In other words, j is a pointer variable containing the address of i (i.e. 2002).
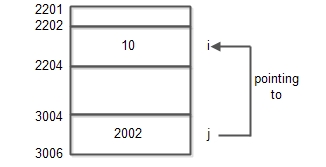
We’ll be covering the following topics in this tutorial:
Declaring Pointer Variable
A pointer variable declares in the same way as that of a normal variable except that the name of the pointer variable must be preceded by an asterisk (*). The syntax for declaring the pointer variable is
data_type *ptr_var_name;
Where data_type represents the data type to which the pointer variable ptr_var_name will be pointing. It may be of basic data types (int, char, float etc.) or user-defined data types (class etc.) The variable ptr_var_name follows the same rule as that of an identifier. The asterisk(*) informs the compiler that the ptr_var_name is not a normal variable; instead, it is a pointer variable. For example
The above declaration states that ptr is a pointer variable that can store any variable with data type int. It cannot point to any other type of variables. Some valid declarations are
In the above declaration of pointers, all the pointer variables occupy the same space in memory, even if they intended to point to different data types. A pointer generally occupies 2 or 4 bytes depending upon the compiler and architecture of the CPU.
You can declare the pointer variable and the declaration of normal variables provided the data type of each of them is the same.
For example:
int *ptr ,a,b;
Declares a pointer variable ptr and two int variables a and b, respectively.
Initialization of Pointer Variables
Once the pointer variable is declared, it must be properly initialized before it can be used.
For example, in the statement.
int *ptr;
Pointer variable ptr doesn’t point to anything. If used, the uninitialized pointer will not cause any compile-time error as that of normal variables but, if used, give inappropriate results. The pointer variable is of no use until it contains the address of some other variable. For this, C++ provides two operators to work, especially with pointers.
• Address of operator(&)
• Indirection operator(*)
Address of Operator Or Reference Operator(&)
We can initialize the pointer variable by assigning the address of some other variable. The address of the variable can be obtained by preceding the name of the variable with the address of the operator(&). This operator returns the address of the variable. For example,
int i=5; int *ptr = &i;
In the second statement, pointer variable ptr is initialized with the address of variable i. We can also define the variable i and initialize the pointer variable ptr with the address of variable i in a single statement provided the variable i is defined before ptr.
int i=5,*ptr = &i;
#include<iostream.h> #include<conio.h> int main() { clrscr(); int i,*ptr; i = 5; ptr=&i;//stores address of i cout<<"Value of i="<<i; cout<<"\nAddress of i="<<&i; cout<<"\nValue of ptr="<<ptr; getch(); return 0; } Output : Value of i = 5 Address of i = 2202 (supposed,may vary) Value of ptr = 2202
Explanation: In the above program, pointer variable ptr stores the address of variable i. Then value and address of i along with the value of ptr are displayed.
Indirection Or Dereference Operator(*)
Once the pointer variable stores the address of some variable, it can be used to indirectly access the value of the variable whose address is stored in it. For this, precede the pointer variable with an indirection operator (*). It is also known as dereference operator as it returns the value of the variable pointed to by the pointer variable.
#include<iostream.h> #include<conio.h> int main() { clrscr(); int i = 5,*ptr; ptr=&i; cout<<"Value stored at address of ptr = "<<*ptr; getch(); return 0; } Output : Value stored at address of ptr 5
Explanation: Since the pointer variable ptr contains the address of i, so *ptr will return the value at the address stored in ptr (i.e. the value of i).
Whenever you perform any operation on the dereference pointer, the resultant changes are always applied to the value whose address is stored in it. So, the statement.
*ptr = 10;
Will assign 10 to the variable i, which is pointed to by the variable ptr. The dereference pointer can also be used to receive the input value from the user. For example
cin>>*ptr;
#include<iostream.h> #include<conio.h> int main() { clrscr(); int i=5,j=2,*ptr; ptr=&i; cout<<''Address of i="<<&i; cout<<"\nValue stored in ptr="<<ptr; cout<<"\nValue pointed by ptr="<<*ptr; *ptr=10; cout<<"\nValue of i= "<<i; j = *ptr; cout<<"\nValue of j="<<j; getch(); return 0; } Output : Address of i=2202 Value stored in ptr = 2202 Value pointed by ptr = 5 Value of i = 10 Value of j = 10
Explanation: In the above program, i and j are the integer variables. Initially, i and j contain values 5 and 2, respectively.
The statement
ptr=&i;
Assigns the address of i to pointer variable ptr and then displays the value stored in the memory location pointed to by ptr.
The statement
*ptr = 10;
Assigns the value 10 to the variable whose address is stored in ptr (i.e. i).
Then, the statement
j = *ptr;
Assigns the value 10 to j.