A while loop in Python is a control flow statement that repeatedly executes a block of statements based on a given boolean condition. In this tutorial, you will learn how to create a while loop in Python.
We’ll be covering the following topics in this tutorial:
What is while loop in Python?
While loop in Python uses to iterate over a block of code as long as a given expression evaluates to (boolean) “true.” The block stops execution if and only if the given condition returns to be false. The while loop can be considered as a repeating if statement. It is also known as a pre-tested loop.
We usually use this loop when we do not know how many times to iterate before.
While Loops versus For Loops
In Python, the ‘while’ and for’ loops are very similar and confusing. Remember, the for’ loop repeats for a default number of times according to the sequence’s length. On the other hand, a ‘while’ loop will continue to run as long as the condition is true.
Therefore, when a repeated operation is required, you should use the “while” loop, but you do not know beforehand how many iterations are required.
Syntax of while Loop in Python
The syntax of a while loop in Python programming language is
while expression:
statement(s)
In the while loop, statement(s) may be a single statement or a block of statements. The loop iterates as long as the situation is true.
This process continues until the False expression evaluated, the program control immediately passes to the line after the loop.
Flowchart of while Loop
1. First, the conditions are checked. If the condition returns false, the loop is terminated, and the control jumps into the program’s next statement following the loop.
2. If the condition is true, the statements within the loop are executed, and then the control jumps to the start of the loop for the next iteration.
These two steps repeatedly happen as long as the condition specified in the while loop is true.
Python – While loop example
Here is an example of a while loop. In this example, we have a variable num, and we are displaying the value of num in a loop. The loop has an increase in operation in which we increase the num value. It is an extremely important step. The while loop must be increased or decreased; otherwise, the loop will run indefinitely.
The variable num initialized to 0 before the block starts. Till it is less than 5, num is incremented by one and printed to display the numbers’ sequence, as below.
num = 0 #loop will repeat itself as long as #num < 5 remains true while num < 5: print("num =", num) #incrementing the value of num num = num + 1
Output:
Infinite while loop
num = 1 while num<5: print(num)
Loop will print ‘1’ indefinitely because we don’t update the value of num within the loop. The value of num always stays 1, and the condition num < 5 returns true at all times.
Nested while loop in Python
If a while loop is present within a while loop, it is called a nested while loop. Let’s take an example of this concept to understand.
loop_a = 1 loop_b = 5 while loop_a < 4: while loop_b < 8: print(loop_a, ",", loop_b) loop_b = loop_b + 1 loop_a = loop_a + 1
Output:
Python – while loop with else block
We can have an ‘else’ block linked to the while loop. The block ‘else’ is optional. It only executes after the execution of the while loop.
num = 10 while num > 5: print("num =", num) num = num - 1 else: print("loop is ended")
Output: 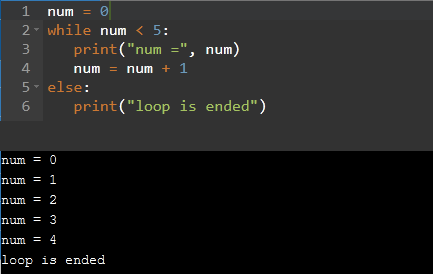