Strings often used in programming. Most of the programs use strings such as names, addresses, messages and many more. A string is a sequence of Unicode characters. Unlike most of the programming languages such as C/C++ where strings treated as an array of characters, Java treats a string as an object. Java provides the following classes for storing and processing strings.
• String
• StringBuffer
• StringBuilder
All these classes defined in java.lang package so they are accessible to all your programs by default.
String Class in java
The java.lang.String class is used to bring a String object(s). String objects are immutable (read-only), i.e. once the String object has created, there is no way to modify the string of text it represents.
You can construct a String object implicitly or explicitly.
Implicit Creation of String Object
A String object can be created implicitly by using a string literal in the same way as you initialise variables of primitive types. For example:
String str = “Welcome to Java”;
When the Java compiler encounters this statement, it creates a String object with the contents Welcome to Java and assigns its reference to string reference variable str.
Since strings are immutable so if we write the statement,
str = “Good Morning”;
the new String object with the contents Good Morning will be created and its reference is_assigned to str. The String object with contents Welcome to Java still exists after this assignment, but it is no longer referenced by str.
Another way of implicit conversion is by using the + or += operator on two String objects to create a new one. For example :
String s1 = “Hello”;
String s2 = ” World”;
String s3 = s1+s2;//Results in Hello World
Consider another example
String s4 = “Good”;
s4+ = ” Morning”;//Results in good Morning
Explicit Creation of String Object
The String object can be created explicitly by using the new keyword and a constructor in the same way as you have created objects in previously. For example The statement
String str = new String(“Welcome to Java”);
Creates a String object initialised to the value of the string literal “Welcome to Java” and assigns a reference to string reference variable str. Here, String (“Welcome to Java”) is actually a constructor of the form String (string literals). When this statement compiled, the Java compiler invokes this constructor and initialises the String object with the string literal enclosed in parentheses which passed as an argument.
You can also create a string from an array of characters. To create a string initialised by an array of characters, use the constructor of the form
String (charArray)
For example, consider the following character array.
char[] charArray ={‘H’,’i’,’ ‘,’D’,’I’,’N’,’E’,’S’,’H’};
If we want to create a String object, str1 initialised to value contained in the character array chrArr, then use the following statement,
String str1 = new String(chrArr);
One should remember that the contents of the character array are copied, subsequent modification of the character array does not affect the newly created string.
In addition to these two constructors, String class also supports the following constructors,
• String (): It constructs a new String object which is initialized to an empty string (” “). For example:
String s = new String();
Will create a string reference variable s that will reference an empty string.
• String (String strObj): It constructs a String object which is initialized to same character sequence as that of the string referenced by strObj. For example, A string reference variable s references a String object which contains a string Welcome On execution of the following statement
String s2 = new String(s);
The string reference variable s2 will refer to a new String object with the same character sequence Welcome in it.
• String (char [] chArr, int startIndex, int count): It constructs a new String object whose contents are the same as the character array, chArr, starting from index startlndex upto a maximum of count characters. For example: Consider the following statements
char[] str = {‘W’,’e’,’l’,’c’,’o’,’m’,’e’};
String s3 = new String(str,5,2);
On execution, the string reference variable s3 will refer to a string object containing a character sequence me in it.
• String (byte [] bytes): It constructs a new String object by converting the bytes array into characters using the default charset. For example, consider the following statements,
byte[] ascii ={65,66,67,68,70,71,73};
String s4 = new string (ASCII);
On execution, the string reference variable s4 will refer to a String object containing the character equivalent to the one in the bytes array.
• String (byte [] bytes, int startIndex, int count): It constructs a new String object by converting the bytes array starting from index startIndex upto a maximum of count bytes into characters using the default charset. For example: On executing the statement,
String s5 = new String(ascii,2,3);
The string reference variable s5 will refer to a String object containing character sequence CDE.
• String (byte [] bytes, String charsetName): It constructs a new String object by converting bytes array into characters using the specified charset.
• String(byte[] bytes, int startIndex, int count, String charsetName): It constructs a new String object by converting the bytes array starting from index startIndex upto a maximum of count bytes into characters using the specified charset.
• String (StringBuffer buffer): It constructs a new String object from a StringBuffer object.
Now let us consider an example to explain how these constructors are used.
strl Welcome to Java
str2 Hi DINESH
str3 Ra
str4 ABCDEFG
strS CDE
str6 =
str7 = Welcome
str = Welcome Dinesh Thakur
String Class Methods in Java
The String class provides us with numerous methods. These methods help us to access individual characters of the string, compare strings, search string, extract substrings and so on. These methods are divided into the following:
• Determining string length, characters and combining strings
• Searching strings
• Comparing strings
• Converting, replacing, splitting and matching strings
• Obtaining substrings
• Conversion between strings and arrays
• Obtaining the characters in a string as an array of bytes
While discussing all these methods, we assume that the strings st, s2 and s3 exists which are defined as follows,
String s1 = “Welcome”;
String s2 = “Welcome”;
String s3 = “Where there is a will, there is way”;
Getting String Length, Characters And Combining Strings
The String class provides methods for obtaining length, retrieving individual characters and concatenating strings. These include
• length()
• charAt()
• concat()
• int length (): This method returns the number of characters in a string. For example: The statement,
int k = s1.length();
will store 7 in a variable k i.e. the number of characters in the String object referenced by s1 (i.e. Welcome).
• char charAt (int index): This method returns the character in the string at the specified index position. The index of the first character is 0, the second character is 1 and that of the last character is one less than the string length. The index argument must be greater than or equal to 0 and less than the length of the string. For example :
s1.charAt(1)
will return character ‘e’ i.e. character at index position 1 in the string referenced by s1.
• String concat (String str): This method concatenates the specified string to the end of the string. For example : The statement,
s1.concat(“Everybody”);
will concatenates the strings ‘Welcome’ and ‘Everybody’.
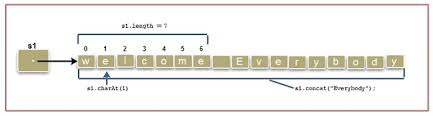
Searching Strings
Searching a string for finding the location of either a character or group of characters (substring) is a common task. For example: In some situations, usernames and passwords are stored in a single string in which they are separated from each other by a special character such as the colon (Example: username: password). To get the username and password separately from such a string, there must be some method such that we can search the string and return the location of the colon character. The String class provides the following methods for performing these tasks,
• indexOf();
• lastIndexOf();
• Int indexOf (int ch): This method returns the index position of the first occurrence of the character ch in the string. If the specified character ch is not found within the string then -1 is returned. For example:
The statement, s1.indexOf(‘e’) will return 1 as index starts from 0.
Another version of this method is int indexOf (String str)
It is same as above except that it accepts a string argument. For example,
s3.indexOf(“there”)
Will return 6, i.e. the index position of the first occurrence of substring “there” in the string.
• Int indexOf (int ch, int fromIndex): This method is same as the previous method, but the only difference is that the search will start from the specified index (i.e. fromIndex) instead of 0. For example, s1. indexOf (‘e’ ,2) will return 6 as searching will start from index 2.
Another version of this method is int indexOf (String str, int fromIndex)
It is same as above except that it accepts a string as the first argument instead of a character.
For example, The statement s3.indexOf (“there”, 8) will return 23.
The lastIndexOf() method also has four versions as that in case of indexOf() method. The four versions of lastIndexOf() method have the same parameters as the four versions of the indexOf() method. The difference is that this method locates the last occurrence of the character or substring in the string depending upon the argument passed. This method performs the search from the end of the string towards the beginning of the string. If it finds the character or substring, the index is returned. Otherwise, it returns-1.
For example, consider the following statements.
s1.lastIndexOf(‘e’) // returns 6
s2.lastIndexOf(‘e’,3) // returns 1
s3.lastIndexOf(“there”) // returns -23
s3.lastIndexOf(“there”,20) // returns 6
s3.lastIndexOf(“Hi”,5) // returns -1
str.indexOf(‘e’,3) = 6
str.indexOf(\Thakur\”) = 3
str.indexOf(\Thakur\”,4) -1
str.lastlndexOf(‘e’) = 6
str.lastlndexOf(‘e’,3) = 1
str.lastlndexOf(\”Thakur\’) = 3
str.lastlndexOf(\Thakur\”,4) 3
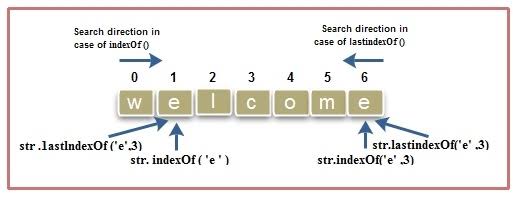
Comparing Strings
The String class also provides some methods for comparing strings. While discussing these, we assume string s1 contains ‘Welcome’ in it.
• boolean equals (String str): This method checks whether the contents of the String object referenced by str is equal to the contents of a String object through which this method is invoked. It returns true if the strings are equal and false otherwise. For example:
s1.equals(“Welcome”) // returns true
s1.equals(s2) // returns false
• boolean equalslgnoreCase (String str): It is same as above except it ignores the case of the characters in the string (i.e. treats uppercase and lowercase characters to be equivalent). For example,
s1.equalslgnoreCase(“welcome”) // returns true
s1.equalslgnoreCase(s2) // returns true
• int compareTo (String str): This method compares the contents of String object referenced by str with the contents of invoking string by comparing the successive corresponding characters, starting with the first character in each string. The process continues until either the corresponding characters are found to be different or the last character in one or both strings is reached. Characters are compared lexicographically (i.e. according to their Unicode representation). This method returns
• 0 if the two strings are equal, i.e. they contain the same number of characters, and the corresponding characters are identical.
• A value greater than 0 if the invoking string has a character greater than the corresponding character in the String object referenced by str.
• A value less than 0 if the invoking string has a character less than the corresponding character in the String object referenced by str. For example:
s1.compareTo(“Welcome”) // returns 0
s1.compareTo(s2) // returns-32
s2.compareTo(s1) // returns 32
NOTE: A syntax error will occur if you compare strings using comparison operators >, >=, <=, <.
• int compareTolgnoreCase (String str): This method is same as compareTo() method except that it ignores the case of string characters. For example:
s1.compareTolgnoreCase (s2) returns 0.
• boolean startsWith (String str): This method determines whether the invoking string starts with the specified string str. If so, the method returns true otherwise returns false. For example,
s1.startsWith(“We”) //returns true
s1.startsWith(“Well”) //returns false
• boolean startsWith(string str, int startIndex): It is similar to the previous method, but it tests whether the invoking string starts with the specified string str beginning at a specified index, startlndex. For example,
s1.startsWith(“We”,2) // returns false
• boolean endsWith (String str): This method determines whether the invoking string ends with the specified string str or not. If so the method returns true otherwise returns false. For example:
s3.endswith(“Java”) // returns true
s1.endswith (“come”) // returns false
• boolean regionMatches(int startlndex, String str, int strStartlndex, int len): This method checks whether a region (portion) of the invoking string matches the specified region of the specified String object referenced by str. The parameter startIndex specifies the starting index in the string that invokes the method. The parameter str is the comparison string, and the parameter strStartlndex specifies the starting index in the string str. The last parameter len specifies the number of characters to compare between two strings. It returns true if the specified number of characters are lexicographically equivalent. For example,
s1.regionMatches(2,”come”,1,3) // returns true
• boolean regionMatches(boolean ignoreCase, int startlndex, String str, int strStartlndex, int len): It is similar to the above method except that it will ignore case if the value in the first parameter ignoreCase is true. For example,
s1.regionMatches(true,2,”COME”,1,3) // returns true.
Now let us consider an example to shows how these work.
str.equalsIgnoreCase(\”welcome\”) = true
str.compareTo(\”welcome\”) = -32
str.compareToIgnoreCase(\”welcome\”) = 0
str.startsWith(\”We\”) = true
str.startsWith(\”We\”,2) = false
str.endsWith(\”me\”) = true
str.regionMatches(4,\”COME\”,1,3) = false
str. regionMatches (true, 4, \”come\”, 1,3) = true
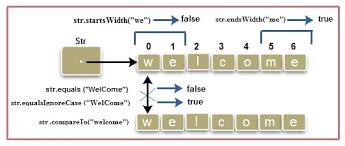
Converting, Replacing, Splitting and Matching Strings
The String class provides methods for converting, replacing, splitting and matching strings. These include
• String toLowercase (): This method converts all of the characters in the string to lowercase. For example,
“Hello”.toLowercase(); //returns hello
• String toUppercase (): This method converts all of the characters in the invoking string to uppercase. For example,
s1.toUppercase(); //returns WELCOME
• String trim (): This method returns a copy of the string, with leading and trailing white spaces omitted. For example,
String s1 =” Good Morning “;
s1.trim(); //returns Good Morning
• String replace (char oldchar, char newchar): This method returns a new string resulting from replacing all the occurrence of oldchar in the invoking string with newchar. For example,
s1.replace(‘e’, ‘E’); //returns Welcome
•String replaceAll (String regeX, String replace): This method replaces all substrings in the invoking string that matches the given regular expression pattern regeX with the specified replacement string replace. For example,
s1.replaceAll(“[aeiou]”,”#”);
It will replace all vowels in the invoking string with # symbol. Here [aeiou] is a regular expression which means any of the characters between the square brackets will match
s3.replaceAll(“is”,”was”);
This statement will replace the substring is with the substring was in the given string.
• String replaceFirst (String regex, String replace): This method replaces the first substring in the invoking string that matches the given regular expression with the specified replacement string replace. For example,
s3.replaceFirst(“is”,”was”);
It will replace the first occurrence of substring is with the substring was in the given string.
• String [] split (String regex): This method splits the invoking string into substrings at each match for a specified regular expression regex and returns a reference to an array of substrings. For example, consider the statements.
String str = “Welcome to Java”;
String[] s = str.split(“[]”);
The first statement defines the string to be analyzed. The second statement calls the split () method for the str object to decompose the string. The regular expression includes the delimiter space so it will return a reference to array of strings where s[O] contains Welcome, s[1] contains to and s[2] contains Java.
Now let us consider a program that explain these operations.
This method tests whether or not the invoking string matches the given regular expression regex.
It returns true if invoking string matches the given regular expression otherwise it returns false.
It is different from the equals () method where you can only use the fixed strings. For
example,
“Javadoc”.matches(“Java”) // returns false
“Javadoc”.matches(“Java.*”) // returns true
Obtaining Substrings
Programmers often need to retrieve an individual character or a group of characters (substring) from a string. For example: In a word processing program, when a part of a string is copied or deleted. While charAt () method returns only a single character from a string, the substring () method in the String class can be used to obtain a substring from a string.
• String substring(int beginIndex): This method returns a new string that is a substring of the invoking string. The substring returned contains a copy of characters beginning from the specified index beginIndex and extends to the end of the string. For example,
s1.substring(3); // returns substring ‘come’
• String substring (int beginIndex, int endIndex): This method is another version of the previous method. It also returns a substring that begins at the specified begin Index and extends to the character at index endIndex-1. For example,
s1.substring(3,6); // returns substring “come”.
s1.substring(3,6) = com
Explanation: The Below Fig depicts the use of substring () method.
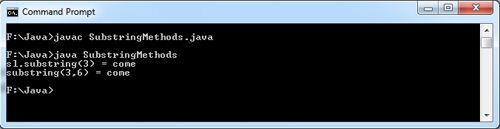
Conversion Between String and Arrays
As we know that strings are not arrays, so the String class also provides methods to convert a string to an array of characters and vice versa. These include
• char [] toCharArray (): This method converts the invoking string to an array of individual characters. For example The statement,
char[] ch = s1.toCharArray();
will convert the string Welcome which is referenced by s1 to an array of characters such that ch [0] contains ‘W,’ ch [1] contains ‘e’ and so on.
• void getChars(int srcBegin, int srcEnd, chsr[] dest, int destBegin): This method copies a substring of the invoking string from index srcBegin to index srcEnd-1 into a character array dest starting from index destBegin. For example The statement,
char[] txtArray = new char[3];
s1.getChars(3,6,txtArray,0);
will copy characters from the String object slat index position 3 to 5 (i.e. 6 – 1) inclusive, so txtArray[0] will be ‘c’, txtArray[1] will be ‘0’ and txtArray[2] will be ‘m’.
• static String valueOf (char [] ch): This method will covert an array of characters to a string. For example : The statements,
char[] ch = {‘W’, ‘E’, ‘L’, ‘C’, ‘O’, ‘M’, ‘E’};
String str String.valueOf(ch);
will return the string Welcome from a character array ch and store the reference in str. There are several versions of valueOf () method that can be used to convert a character and numeric values to strings with different parameter types including int, long, float, double and char.
Now let us consider an example to show how these work.
ch [1] e
ch [2] 1
ch [3] c
ch [4] 0
ch [5] m
ch [6] e
txtArray[0] = c
txtArray [1] = 0
txtArray[2] = m
str = Welcome
Obtaining Characters in a String as an Array of Bytes
The String class also provide methods for extracting characters from a string in an array of bytes. This is achieved using the getBytes () method in the String class. This method converts the original string characters into the character encoding used by the underlying operating system which is usually ASCII. For example : The statement,
byte[] txtArray = s1.getBytes();
extracts character from the String object s1 and stores them in the byte array txtArray. There are many other forms of getBytes () available.
Now let us consider an example,
txtArray[1] = 101
txtArray[2] = 108
txtArray[3] = 99
txtArray[4] = 109
txtArray[5] = 111
txtArray [6] = 101