In this tutorial, We will check a given positive integer N. The task is to write a C program to check if the number is prime or not.
We’ll be covering the following topics in this tutorial:
What is Prime number in C?
Prime number in C: A Prime number is a positive integer that is greater than one and divided only by one and itself. For example, {2, 3, 5, 7, 11, 13, 17, 19, 23} are the prime numbers.
To check whether the number is prime or not iterate through each of the numbers starting from 2 to sqrt(N) with a for loop and check if it divides N. When we find any number that divides, then we return false. If we didn’t see any number between 2 and sqrt(N) which divides N, then it implies that N is prime and we’ll return True. For example
3 = 1 * 3
Some prime number are − 1, 2, 3, 5 , 7, 11 etc.
Algorithm for Prime Number
START
Step 1 → Enter variable V
Step 2 → Divide the Number by V-1.
Step 3 → If V is divisible by (V-1 to 2) it is not prime
Step 4 → Else it is prime
STOP
Pseudocode for Prime Number
procedure prime_number : number FOR loop = 2 to number - 1 check if number is divisible by loop IF divisible RETURN "NOT PRIME" END IF END FOR RETURN "PRIME" end procedure
C Program to Check Prime Number
//prime number program in c #include<stdio.h> int main() { int number, loop, flag = 0; number = 19; for (loop = 2; loop <= number / 2; ++loop) { // condition for non-prime if (number % loop == 0) { flag = 1; break; } } if (number == 1) { printf("1 is neither prime nor composite."); } else { if (flag == 0) printf("%d is a prime number.", number); else printf("%d is not a prime number.", number); } return 0; }
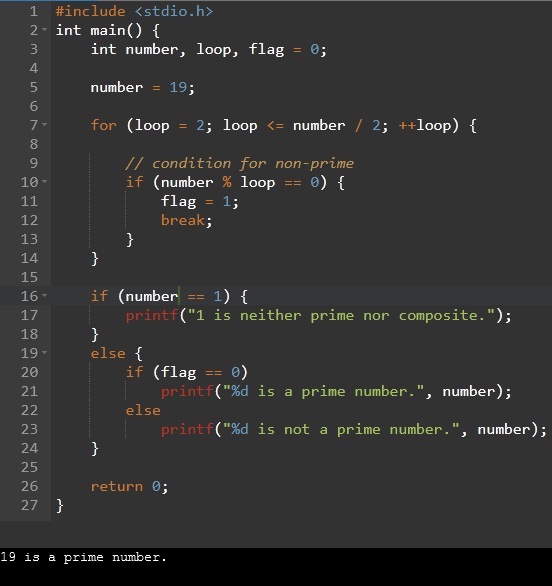