In C, a string is a sequence of characters terminated by a null character (‘\0’). Strings can create using string-literals, which are sequences of characters with double quotation marks; for example, a string of literal “Computer Notes.”
The C library offers a wide array of functions for string operations.
Functions | Description |
strcat() | Function to concatenate(merge) strings. |
strcmp() | Function to compare two strings. |
strcpy() | Function to copy a string to another string. |
stricmp() | Function to compare two strings ignoring their case. |
strlen() | Function to calculate the length of the string. |
strlwr() | Converts the given string to lowercase. |
strrev() | Function to reverse the given string. |
strupr() | Converts the given string to uppercase. |
strdup() | Duplicates a string. |
strnicmp() | Compares the first n characters of one string to another without being case sensitive. |
strncat() | Adds the first n characters at the end of second string. |
strncpy() | Copies the first n characters of a string into another. |
strchr() | Finds the first occurrence of the character. |
strrchr() | Finds the last occurrence of the character. |
strstr() | Finds the first occurrence of string in another string. |
strset() | Sets all the characters of the string to a given character. |
strnset() | Sets first n characters of the string to a given character. |
We’ll be covering the following topics in this tutorial:
The strlen() Function
Syntax : size_t strlen(const char *len);
The strlen() function is one of the inbuilt string function in c programming. It takes one argument len, which can be a string constant or a string variable. It returns the length of the string stored in the array len, i.e., the number of characters in the string excluding the null character(‘\0’). If the string is empty, it returns zero.
Step by Step working of the below Program Code:
START
Step 1 → To use strlen(), we need to declare #include<string.h> header file.
Step 2 → strlen() accepts only one parameter.
Step 3 → strlen() returns the number of string characters.
STOP
To Calculate the length of the string using library function strlen()
#include<stdio.h> #include<string.h> int main() { char len[] = "Computer Notes"; printf("Length of String = %d ", strlen(len)); return 0; }
The strcat() Function
The strcat( ) function appends the src string at the end of the dest string, i.e., character arrays as arguments of which first argument dest is a string variable. The second argument src can be a string variable or string constant. This function appends a copy of src to the end of dest. The first character of src overwrites the terminating null character (‘\0’) of dest. The length of the resulting string is strlen(dest)+ strlen(src). In addition to appending string src to string dest, this function also returns dest. Its syntax is as follows,
Syntax : char *strcat(char *dest, const char *src);
For example, the following program reads two strings of less than 100 characters and uses strcat() to combine them and bring the resulting string into an array.
Step by Step working of the below Program Code:
START
Step 1 → To use strcat(), we need to declare #include<string.h> header file.
Step 2 → strcat() accepts two parameters.
Step 3 → Both parameters must be a string.
STOP
#include <stdio.h> #include<string.h> int main() { char dest[100] = "Computer ", src[30] = "Notes"; strcat (dest, src); printf("Combined Strings are : %s ", dest); return 0; }
The strcmp() Functions
Syntax : int strcmp(const char *lhs, const char *rhs);
The strcmp() function compares two null-terminated character arrays lexicographically. The strcmp ( ) function compares string lhs with the contents of string rhs by comparing successive corresponding characters, starting with the first character in each string. This process continues until either the corresponding characters are formed to be different, or the last character in one or both strings is reached.
If strings are the same, strcmp() returns 0. If the first string is shorter than the second, strcmp() returns a negative value, while if it is larger, it returns a positive value.
if lhs < rhs returns a value < 0
if lhs == rhs returns 0
if lhs > rhs returns a value > 0
A string shorter than another if one of the following conditions is true:
1. The first n character of the string matches, but in the first string the value of the n+1 string is shorter than in the second string.
2. All character matches, but the first string is shorter than the second.
For example, the strcmp(“Notes,” “notes”) statement returns a negative value because the first non-matched ASCII code ‘N’ is lower than the ASCII code of ‘n.’ The strcmp(“w,” “more”) statement returns a positive value because the initial non-matched ‘w’ code in ASCII is larger than the ‘m’ ASCII code.
We’ll implement the strcmp() function, to show you an implementation.
#include <stdio.h> #include <string.h> int main(void) { char lhs[100] = "Notes", rhs[100] = "notes"; int result; result = strcmp(lhs, rhs); if(result == 0) printf("The strings dest and src are same "); else { printf("Different texts\n"); } return 0; }
The strcpy() Functions
The strcpy ( ) function takes two arguments, of which first argument ‘dest’ is a string variable and second argument ‘src’ can be a string variable or string constant. It copies the contents of string ‘src‘, including the null character(‘\0’), replacing what was previously stored in the string ‘dest’.
You can use the == operator to copy integers, However, you can’t use the = operator to copy the strings. Strings in C are represented as arrays of characters with a terminating null-character, so using the = operator will only save the address (pointer) of a string.
The = operator can be used to copy integer, However, you can’t use the = operator to copy the strings. Strings in C represent character arrays with a terminating null character, so the = operator saves a string address.
For example,
char str[10];
str = "Notes"; /* Wrong, since str is an array. */
In fact, strcpy() function is available in string.h to copy strings. Sufficient space must be allocated before
copying to the destination.
Syntax : char *strcpy(char *dest, const char *src);
strcpy() copies the src string into the dest memory. After copying the null character, strcpy() ends and returns the dest pointer. strcpy() can’t modify the string since src declare as const. The strcpy() and strncpy() behavior are undefined when the source and destination strings overlap. In the example below, strcpy() copies “Notes” in str.
char str[10];
strcpy(str, "Notes");
The following program reads and uses strcpy() to copy a string of less than 100 characters in an array:
#include <stdio.h> #include <string.h> int main(void) { char lhs[100], rhs[100]; printf("Enter text: "); fgets(rhs, sizeof(rhs), stdin); strcpy(lhs, rhs); printf("Copied text: %s\n", lhs); return 0; }
The strchr(), strrchr() Functions
The functions strchr and strrchr find a character in an array of characters that is NUL-terminated. Strchr returns the first occurrence pointer and strrchr the last occurrence.
Syntax : char *strrchr(const char *src, int chr);
It scans a string src in the reverse direction, looking for a specific character chr.
#include <stdio.h> #include<string.h> int main() { char src[] = "Computer Notes", chr = 't'; char *toSearchFor; toSearchFor = strchr(src, chr); if(toSearchFor) printf("First position of : %s\n", toSearchFor); toSearchFor = strrchr(src, chr); if(toSearchFor) printf("Last position of : %s", toSearchFor); return 0; }
Outputs :
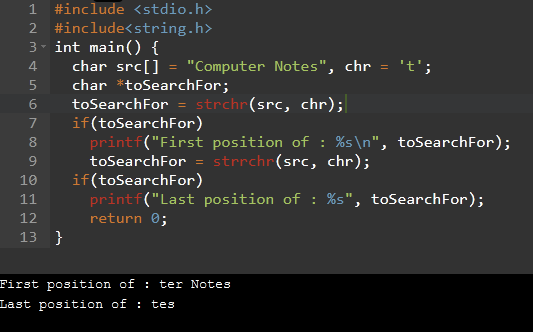
The stricmp() Function
The stricmp() is one of the c programming string function in which two strings are compared, without distinction between uppercase and lowercase letters. If the strings are the same, 0 comes back. Otherwise, a non-zero value will return.
Syntax : stricmp(dest, src);
Step by Step working of the below Program Code:
START
Step 1 → To use stricmp(), we need to declare #include<string.h> header file.
Step 2 → stricmp() accepts two parameters.
Step 3 → Both parameters must be a string.
STOP
#include <stdio.h> #include <stdlib.h> #include <string.h> int main(void) { char dest[20] = "Computer Notes"; char src[20] = "COMPUTER NOTES"; int n; n = stricmp(dest, src); if( n == 0) printf("The strings are same "); else if(n == -1) printf("The string dest is lesser than src"); else printf("The string dest is greater than src"); return 0; }
The strlwr() Function
The strlwr() is one of the c programming string functions used to convert the UPPERCASED characters to lowercase characters. It takes one argument str, which is a string variable. It takes the following form,
Syntax : strlwr(src);
After converting the string into lowercase, it stores the result back in string str and returns string str. Consider an example where the contents of string variable str is “HELLO” then the function call strlwr(str); result in value “hello” being assigned to str.
Step by Step working of the below Program Code:
START
Step 1 → To use strlwr(), we need to declare #include<string.h> header file.
Step 2 → strlwr() accepts one parameters.
Step 3 → Parameter must be a string.
STOP
#include <stdio.h> #include<string.h> int main() { char src[50] = "COMPUTER NOTES"; printf(" %s ", strlwr(src)); return 0; }
The strrev() Function
The strrev( ) function is one of the c programming string function which is used to reverse a given string, except the terminating null character. It returns the pointer to a reversed string. So on using this function on the string “hello,” we get “olleh”.
Syntax : strrev(src);
Step by Step working of the below Program Code:
START
Step 1 → To use strrev(), we need to declare #include<string.h> header file.
Step 2 → strrev() accepts one parameters.
Step 3 → Parameter must be a string.
STOP
#include <stdio.h> #include<string.h> int main() { char src[20] = "Notes"; printf(" %s ", strrev(src)); return 0; }
The strupr() Function
The strupr() function is just the reverse of the strlwr () function. It is used to converts the lowercase characters to UPPERCASE characters. It takes one argument str, which is a string variable. Its takes the following form,
Syntax : strupr(src)
Consider an example where the contents of a string variable str is “hello” then function call strupr(str); result in value “HELLO” being assigned to str.
Step by Step working of the below Program Code:
START
Step 1 → To use strupr(), we need to declare #include<string.h> header file.
Step 2 → strupr() accepts one parameters.
Step 3 → Parameter must be a string.
STOP
#include <stdio.h> #include<string.h> int main() { char src[30] = "notes"; printf("The string in uppercase : %s ", strupr(src)); return 0; }
The strdup() Function
strdup() is used to duplicate a given string.
Syntax : strdup(src)
Step by Step working of the below Program Code:
START
Step 1 → To use strdup(), we need to declare #include<string.h> header file.
Step 2 → strdup() accepts one parameters.
Step 3 → Parameter must be a string.
STOP
#include <stdio.h> #include<string.h> int main() { char src[50] = "Computer Notes", *dest; dest = strdup(src); printf("The Original string is : %s ", src); printf("\nThe duplicated string is : %s ", dest); return 0; }
The strnicmp() Function
strnicmp() is used to compare the first n numbers of characters in a string src and dest.
Syntax : strnicmp(src, dest, n);
Step by Step working of the below Program Code:
START
Step 1 → To use strnicmp(), we need to declare #include<string.h> header file.
Step 2 → strnicmp() accepts three parameters.
Step 3 → strnicmp() accepts the first two parameters are string, and the third parameter is an integer.
STOP
#include <stdio.h> #include<string.h> int main() { char src[30] = "Computer Notes", dest[30] = "COMPUTER Notes"; if(strnicmp(src, dest, 8) == 0) printf("The strings src and dest are same "); return 0; }
The strncat() Function
strncat() is used to combine two strings up to a specified length n.
Syntax : strncat(src, dest, n);
Step by Step working of the below Program Code:
START
Step 1 → To use strncat(), we need to declare #include<string.h> header file.
Step 2 → strncat() accepts three parameters.
Step 3 → src is a string which is of character datatype.
Step 4 → dest is also character datatype.
Step 5 → n is a number which is of integer datatype.
Step 6 → Here n describes, how much characters should we need to append from dest to src.
STOP
#include <stdio.h> #include<string.h> int main() { char src[30] = "Computer "; char dest[30] = "Notes"; strncat(src, dest, 5); printf("%s", src); return 0; }
The strstr() Function
strstr() function is used to return the first occurrence of the substring in another string.
Syntax : strstr(dest, src);
On success, strstr returns a pointer to the element in dest where src begins
(points to src in dest).
On error (if src does not occur in dest), strstr returns null.
Step by Step working of the below Program Code:
START
Step 1 → To use strstr(), we need to declare #include<string.h> header file.
Step 2 → strstr() accepts two parameters.
Step 3 → Both parameters are string.
STOP
//strstr() string function in C #include <stdio.h> #include<string.h> int main() { char src[30] = "Computer Notes char str2 [15] = "N"; char *result; result = strstr(src, dest); printf("%s", result); return 0; }
The strset() Function
Strset() is used to set a specific string character. strset() is most commonly used to hide the set of characters while the user uses a password.
Syntax : strset(src, chr);
Step by Step working of the below Program Code:
START
Step 1 → To use strset(), we need to declare #include<string.h> header file.
Step 2 → strset() accepts two parameters.
Step 3 → Fist parameter must be a string whereas second parameter must be a character.
STOP
//strset() string function in C #include <stdio.h> #include<string.h> int main() { char src[30] = "password"; char chr = '*' ; strset(src, chr); printf("String: %s ", src); return 0; }
The strnset() Function
strnset() sets first n characters of a string to a given character.
Syntax : char *strnset(char *src, int ch, size_t n);
#include <stdio.h> #include <string.h> int main(void) { char *src = "ecomputernotes.com"; char ch = '*'; printf("String before strnset(): %s\n", src); strnset(src, ch, 14); printf("String after strnset(): %s\n", src); return 0; }
Output:
String before strnset(): ecomputernotes.com
String after strnset(): **************.com